hw1. pipe() 함수를 사용해 부모 프로세스와 자식 프로세스 간에 대화가 가능한 양방향 통신이 되도록 프로그램을 작성하시오
(Example : parent-쓰기부터)
char msg[256], inmsg[256];
close(fd1[0]);
close(fd2[1]);
while (1) {
fgets(msg, sizeof(msg) - 1, stdin);
write(fd1[1], msg, sizeof(msg) + 1);
read(fd2[0], msg, 255);
}
close (fd1[1]); close (fd2[0]);
while (1) {
read(fd1[0], inmsg, 255);
fgets(inmsg, sizeof(inmsg) - 1, stdin);
write(fd2[1], inmsg, strlen(inmsg) + 1);
}
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
int fd1[2], fd2[2]; // fd1: 부모->자식, fd2: 자식->부모
pid_t pid;
char msg[256];
char inmsg[256];
// 파이프 두 개 생성
if (pipe(fd1) == -1 || pipe(fd2) == -1) {
perror("pipe failed");
exit(1);
}
// 자식 프로세스 생성
pid = fork();
if (pid < 0) {
perror("fork failed");
exit(1);
}
if (pid == 0) { // 자식 프로세스
close(fd1[1]); // fd1의 쓰기 끝단 닫기 (부모가 쓰고 자식이 읽음)
close(fd2[0]); // fd2의 읽기 끝단 닫기 (자식이 쓰고 부모가 읽음)
while (1) {
// 부모로부터 메시지 읽기
read(fd1[0], inmsg, sizeof(inmsg));
printf("Child received: %s", inmsg);
// 부모에게 메시지 보내기
printf("Child: Enter message for parent: ");
fgets(msg, sizeof(msg), stdin);
write(fd2[1], msg, strlen(msg) + 1);
}
} else { // 부모 프로세스
close(fd1[0]); // fd1의 읽기 끝단 닫기 (부모가 쓰고 자식이 읽음)
close(fd2[1]); // fd2의 쓰기 끝단 닫기 (자식이 쓰고 부모가 읽음)
while (1) {
// 자식에게 메시지 보내기
printf("Parent: Enter message for child: ");
fgets(msg, sizeof(msg), stdin);
write(fd1[1], msg, strlen(msg) + 1);
// 자식으로부터 메시지 읽기
read(fd2[0], inmsg, sizeof(inmsg));
printf("Parent received: %s", inmsg);
}
}
return 0;
}
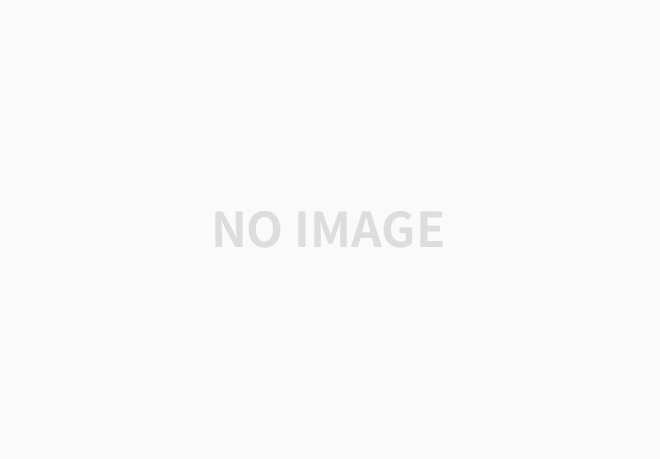
참고 및 출처: 시스템 프로그래밍 리눅스&유닉스(이종원)
'Computer Science > UNIX & Linux' 카테고리의 다른 글
[UNIX/Linux] ep10-1) 메시지 큐, 공유 메모리 (0) | 2024.11.06 |
---|---|
[UNIX/Linux] ep7++) 레코드 락킹(advisory locking) (0) | 2024.11.06 |
CUDA + RAID 기반 데이터 분산 시뮬레이션 (8) | 2024.10.31 |
[UNIX/Linux] ep9) 파이프 (0) | 2024.10.31 |
[UNIX/Linux] ep8+) 시그널 함수 실습 (1) | 2024.10.24 |